Preparing for a React.js Interview at Accenture
If you’re preparing for a React.js interview at Accenture, it’s essential to be well-versed with the core concepts of React, as well as related technologies and best practices. In this blog, we’ll cover some common interview questions you might encounter and provide insights into what interviewers might be looking for.
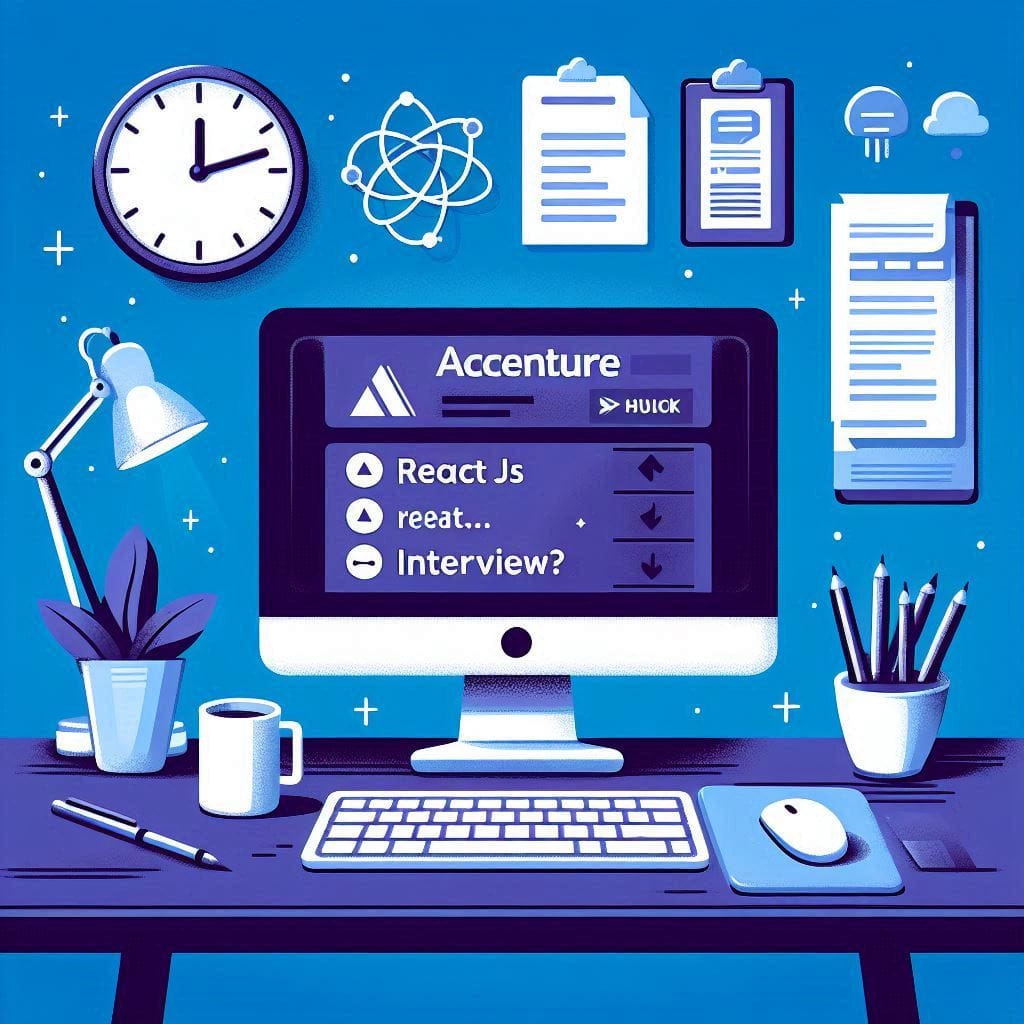
Core React Questions | Beginners
1. What is React?
-React is a JavaScript library for building user interfaces, primarily single-page applications where data changes dynamically over time. It allows developers to create large web applications that can change data, without reloading the page. The main purpose of React is to be fast, scalable, and simple.
2. What are components in React?
– Components are the building blocks of a React application. They are independent, reusable pieces of code that serve as the foundation for building the user interface. Components can be either class-based or functional.
3. What is the difference between functional and class components?
– Functional components are stateless components defined as JavaScript functions. They receive props and return React elements.
– Class components are stateful and defined as ES6 classes. They can manage local state and lifecycle methods.
4. What is JSX?
– JSX stands for JavaScript XML. It is a syntax extension for JavaScript that allows writing HTML directly within React. JSX makes it easier to write and add HTML in React.
5. What are props in React?
– Props (short for properties) are read-only attributes passed from a parent component to a child component. They are used to pass data and event handlers to the child components.
6. What is state in React?
– State is an object that represents the dynamic parts of a component. Unlike props, the state is managed within the component and can be updated over time, usually in response to user actions.
7. Explain the component lifecycle in React.
– React components go through a lifecycle of events:
– Mounting: When the component is being created and inserted into the DOM. Methods include `constructor`, `getDerivedStateFromProps`, `render`, and `componentDidMount`.
– Updating: When the component is being re-rendered as a result of changes to either props or state. Methods include `getDerivedStateFromProps`, `shouldComponentUpdate`, `render`, `getSnapshotBeforeUpdate`, and `componentDidUpdate`.
– Unmounting: When the component is being removed from the DOM. Method includes `componentWillUnmount`.
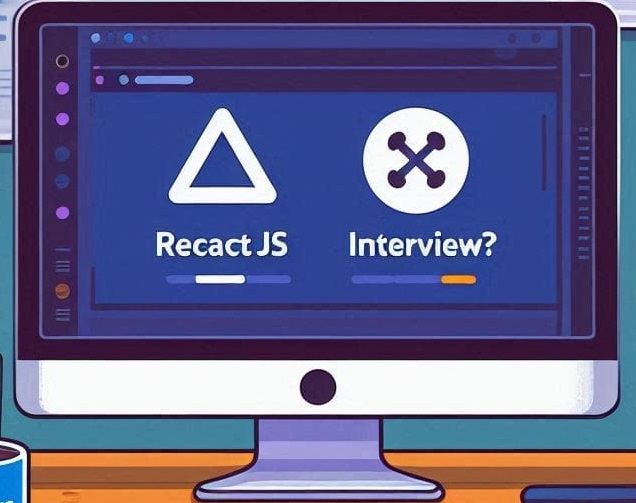
Advanced React Questions | Senior
1. What are hooks in React?
– Hooks are functions that let you use state and other React features in functional components. Common hooks include `useState`, `useEffect`, `useContext`, `useReducer`, and custom hooks.
2. Explain `useEffect` and its use cases.
– `useEffect` is a hook that allows you to perform side effects in functional components, such as fetching data, directly updating the DOM, and setting up subscriptions. It takes two arguments: a function that contains the side effect logic, and an optional array of dependencies that determine when the side effect should be re-run.
3. What is Context API and how is it used?
– The Context API is a way to pass data through the component tree without having to pass props down manually at every level. It is used to share data like themes, user information, and settings across the entire application.
4. What is Redux and how does it work with React?
– Redux is a state management library that provides a centralized store for all the state in an application. It works with React through a series of actions and reducers, allowing components to access and update the global state efficiently.
5. Explain the concept of Higher-Order Components (HOCs)
– HOCs are functions that take a component and return a new component with added functionality. They are used to reuse component logic and are a pattern derived from React’s compositional nature.
### Performance Optimization Questions
1. How do you optimize the performance of a React application?
– Performance optimizations in React can include:
– Using React.memo to prevent unnecessary re-renders of functional components.
– Using `shouldComponentUpdate` in class components.
– Code-splitting and lazy loading components with `React.lazy` and `Suspense`.
– Avoiding inline functions and objects as props.
– Using the production build of React.
– Leveraging useCallback and useMemo hooks for memoizing functions and values.
2. What are React.memo and useCallback?
– `React.memo` is a higher-order component that memoizes a component, preventing it from re-rendering if its props haven’t changed.
– `useCallback` is a hook that returns a memoized version of the callback function, which only changes if one of the dependencies has changed.
### Testing and Tools
1. How do you test React components?
React components can be tested using various tools and libraries such as Jest, React Testing Library, and Enzyme. These tools allow you to write unit tests, integration tests, and snapshot tests.
2. What are some tools you use in your React development workflow?
– Some common tools include:
– Create React App for bootstrapping React projects.
– Webpack and Babel for module bundling and transpiling.
– ESLint and Prettier for code linting and formatting.
– Redux DevTools for debugging state changes in Redux applications.
– Storybook for developing and testing UI components in isolation.
Conclusion
Preparing for a React.js interview at Accenture requires a solid understanding of both basic and advanced React concepts. It’s essential to practice coding problems, understand the inner workings of React, and be familiar with performance optimization techniques and tools. Good luck with your interview preparation!
Feel free to share your own experiences or additional questions you’ve encountered in React.js interviews in the comments below.